Recently I came to know how little many beginner software developers know about Class and Object Relations in Object Oriented Programming. That’s why, I decided to start writing short and manageable articles in regards to Relations Between Objects And Classes.
During my upcoming blog posts, I’ll be focusing on topics such as Dependency, Association, Aggregation, Composition, Implementation and Inheritance.
Starting with Dependency.
#1: What is Dependency?
We are speaking of a Dependency when changes in the definition of class A results to modifications in class B. A dependency between objects occurs when using concrete classes in another class.
For example, when instantiating objects via a constructor method, or specifying input types in method and function signatures.
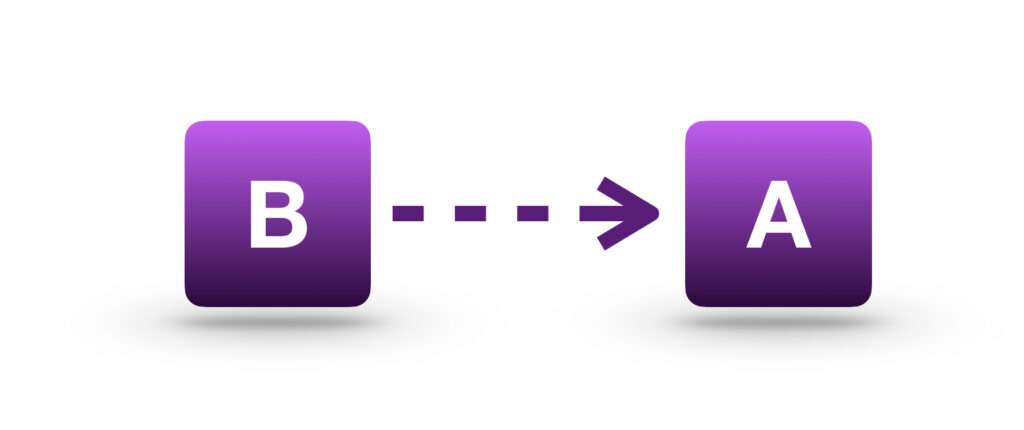
Note: class B can be modified by changes in class A.
A dependency is considered to be the most basic and weakest relations between objects.
Pseudo Code
class Registration is
method signup( _: User): User is
Registration class has a dependency to class User, because its method uses User as input and as a return type.
Note: We can make a dependency even weaker, if we depend our code on Interfaces (protocols ) instead of concrete classes.
interface UserInterface is
name: String
userName: String
password: String
class User implements UserInterface is
name: String
userName: String
password: String
method constructor(String name, String userName, String password) is
this.name = name
this.userName = userName
this.password = password
class Registration is
method signup( _: UserInterface): UserInterface is
Notice how Registration class now has a weaker dependency to the User class because now it uses an interface / protocol .
Swift Code – Dependency Using Concrete Class Name
import Foundation
class User {
let name: String
let userName: String
let password: String
init( name: String, userName: String, password: String) {
self.name = name
self.userName = userName
self.password = password
}
}
class Registration {
func signup( _ user: User ) -> User {
let user = user
return user
}
}
Swift Code – Weaker Dependency Through Using An Interface / Protocol
import Foundation
//Defines a blue print
//for any class that implements this interface
//Multiple class can implement this interface
protocol UserInterface {
var name: String { get set }
var userName: String { get set }
var password: String { get set }
}
//User class implements UserInterface Protocol
class User: UserInterface {
var name: String
var userName: String
var password: String
init( name: String, userName: String, password: String) {
self.name = name
self.userName = userName
self.password = password
}
}
//Registration class has a weaker dependency
//To User class
class Registration {
func signup( _ user: UserInterface ) -> UserInterface {
let user = user
return user
}
}
let user = User(name: "Robert", userName: "rob", password: "yusiyd87434")
let registration = Registration()
let output = registration.signup(user)
print(output.userName) //rob